iOS-App-Security-Class alternatives and similar libraries
Based on the "Security" category.
Alternatively, view iOS-App-Security-Class alternatives based on common mentions on social networks and blogs.
-
CryptoSwift
CryptoSwift is a growing collection of standard and secure cryptographic algorithms implemented in Swift -
RNCryptor
CCCryptor (AES encryption) wrappers for iOS and Mac in Swift. -- For ObjC, see RNCryptor/RNCryptor-objc -
Valet
Valet lets you securely store data in the iOS, tvOS, or macOS Keychain without knowing a thing about how the Keychain works. Itβs easy. We promise. -
UICKeyChainStore
UICKeyChainStore is a simple wrapper for Keychain on iOS, watchOS, tvOS and macOS. Makes using Keychain APIs as easy as NSUserDefaults. -
SwiftKeychainWrapper
DISCONTINUED. A simple wrapper for the iOS Keychain to allow you to use it in a similar fashion to User Defaults. Written in Swift. -
Themis
Easy to use cryptographic framework for data protection: secure messaging with forward secrecy and secure data storage. Has unified APIs across 14 platforms. -
BiometricAuthentication
Use Apple FaceID or TouchID authentication in your app using BiometricAuthentication. -
SwCrypt
RSA public/private key generation, RSA, AES encryption/decryption, RSA sign/verify in Swift with CommonCrypto in iOS and OS X -
SecurePropertyStorage
Helps you define secure storages for your properties using Swift property wrappers. -
KKPinCodeTextField
A customizable verification code textField. Can be used for phone verification codes, passwords etc -
Virgil Security Objective-C/Swift SDK
Virgil Core SDK allows developers to get up and running with Virgil Cards Service API quickly and add end-to-end security to their new or existing digital solutions to become HIPAA and GDPR compliant and more. -
RSASwiftGenerator
Util for generation RSA keys on your client and save to keychain or convert into Data π π -
VoiceItAPI1IosSDK
DISCONTINUED. A super easy way to add Voice Authentication(Biometrics) to your iOS apps, conveniently usable via cocoapods
InfluxDB - Power Real-Time Data Analytics at Scale
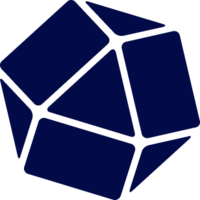
* Code Quality Rankings and insights are calculated and provided by Lumnify.
They vary from L1 to L5 with "L5" being the highest.
Do you think we are missing an alternative of iOS-App-Security-Class or a related project?
README
iOS-App-Security-Class
Simple class to check if iOS app has been cracked, being debugged or enriched with custom dylib and as well detect jailbroken environment
Usage
Just drag SecurityClass.m and SecurityClass.h to your project, then add
#import "SecurityClass.h"
If you want to just test and see how it works, clone repository, open in Xcode and compile.
SecurityClass.m allows you easily check if your iOS App:
- Has been cracked with tool like Clutch or manually
- Is being debugged with 2 different ways to check
- Has been treated with any custom library, for example Cycript or tweaks to crack InApp Purchases
- Is running on jailbroken device
Repository contains example app, feel free to test. If you want to import it in your project just copy SecurityClass.m & SecurityClass.h
This class shouldn't be used unobfuscated, and possibly should be splitted to inline code in desired function related to app security also strings used should be at least encrypted with AES. But for most attackers it will be hard at this point to crack it, even without obfuscation.
Apple FairPlay Crack Detection
Check if currently running binary is encrypted (Signed by developer and Apple) Simply check if app has been treated with tool like Clutch or manually dumped from memory
NSDictionary *resp = [SecurityClass getCurrentBinaryInfo];
NSLog(@"Binary Info:%@",resp); // <- Gives all necessary informations
"Encryption not found" or "cracked" - will appear if app has not been signed by you and/or Apple
Custom dylib injected to memory
Check if any library has been injected into app process(can be easily done on jailbroken device)
bool IfAppContainsDylib = [SecurityClass isDylibInjectedToProcessWithName:@"dylib_name"];
if (IfAppContainsDylib) {
NSLog(@"dylib_name has been injected to app");
} else {
NSLog(@"Not found dylib_name in app");
}
Example - Checking if our app has been attacked with Cycript which uses libcycript.dylib
bool IfAppContainsCycript = [SecurityClass isDylibInjectedToProcessWithName:@"libcycript"];
if (IfAppContainsCycript) {
NSLog(@"libcycript has been injected to app");
} else {
NSLog(@"Not found libcycript in app");
}
Debugger detection
Traditional way for checking if debugger is connected
bool isDebuggerConnected = [SecurityClass isDebuggerConnected];
if (isDebuggerConnected) {
NSLog(@"App is being debugged");
} else {
NSLog(@"Not found debugger");
}
/dev/tty way
bool isDebuggerConnected_tty = [SecurityClass ttyWayIsDebuggerConnected];
if (isDebuggerConnected_tty) {
NSLog(@"App is being debugged /dev/tty");
} else {
NSLog(@"Not found debugger /dev/tty");
}
Proxied Connections
Check if connections between app and server side are being proxied by tools like Charles Proxy For example charles default listening port is 8888 but if necessary all connections can be dropped when proxy is detected.
bool isConnectionProxied = [SecurityClass isConnectionProxied];
if (isConnectionProxied) {
NSLog(@"Connection is being proxied to %@:%@",[SecurityClass proxy_host],[SecurityClass proxy_port]);
} else {
NSLog(@"Connection is not being proxied with http proxy");
}
Jailbroken devices detection
Detect if device is jailbroken, sometimes may detect devices which were jailbroken but no longer are. Can be relatively easily hacked with tools on Cydia which sometimes work sometimes not work to give false result. I suggest using this method along with checking for processes and libraries injected specific for jailbroken device to get more reliable result. Unfortunately from iOS 9 it's not longer possible to get current list of running apps, sysctl now returns 0 for sandboxed environment and other tricks are also blocked by apple due "privacy concerns".
bool isDeviceJailbroken = [SecurityClass isDeviceJailbroken];
if (isDeviceJailbroken) {
NSLog(@"Device is jailbroken");
} else {
NSLog(@"Device is NOT jailbroken");
}