NetClient alternatives and similar libraries
Based on the "Networking" category.
Alternatively, view NetClient alternatives based on common mentions on social networks and blogs.
-
RealReachability
We need to observe the REAL reachability of network. That's what RealReachability do. -
Networking
DISCONTINUED. Easy HTTP Networking in Swift a NSURLSession wrapper with image caching support -
XMNetworking
A lightweight but powerful network library with simplified and expressive syntax based on AFNetworking. -
Digger
Digger is a lightweight download framework that requires only one line of code to complete the file download task -
SOAPEngine
This generic SOAP client allows you to access web services using a your iOS app, Mac OS X app and AppleTV app. -
TWRDownloadManager
A modern download manager based on NSURLSession to deal with asynchronous downloading, management and persistence of multiple files. -
ws โ๏ธ
โ ๏ธ Deprecated - (in favour of Networking) :cloud: Elegantly connect to a JSON api. (Alamofire + Promises + JSON Parsing) -
MultiPeer
๐ฑ๐ฒ A wrapper for the MultipeerConnectivity framework for automatic offline data transmission between devices -
AFNetworking+RetryPolicy
Nice category that adds the ability to set the retry interval, retry count and progressiveness.
WorkOS - The modern identity platform for B2B SaaS
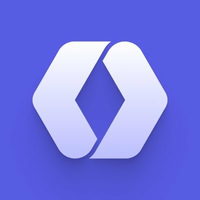
* Code Quality Rankings and insights are calculated and provided by Lumnify.
They vary from L1 to L5 with "L5" being the highest.
Do you think we are missing an alternative of NetClient or a related project?
README
Net is a versatile HTTP networking library written in Swift.
๐ Features
- [x] URL / JSON / Property List Parameter Encoding
- [x] Upload File / Data / Stream / Multipart Form Data
- [x] Download File using Request or Resume Data
- [x] Authentication with URLCredential
- [x] Basic, Bearer and Custom Authorization Handling
- [x] Default and Custom Cache Controls
- [x] Default and Custom Content Types
- [x] Upload and Download Progress Closures with Progress (only iOS >= 11)
- [x] cURL Command Debug Output
- [x] Request and Response Interceptors
- [x] Asynchronous and synchronous task execution
- [x] Inference of response object type
- [x] Network reachability
- [x] TLS Certificate and Public Key Pinning
- [x] Retry requests
- [x] Codable / Decodable / Encodable protocols compatible (JSON / Property List)
- [x] Customizable acceptable status codes range
- [x] watchOS Compatible
- [x] tvOS Compatible
- [x] macOS Compatible
- [x] Alamofire Implementation
- [x] MoyaProvider Extension
- [x] Kommander Extension
- [x] RxSwift Extension
- [x] Stub Implementation
๐ Requirements
- iOS 8.0+ / macOS 10.9+ / tvOS 9.0+ / watchOS 2.0+
- Xcode 9.0+
- Swift 4.0+
๐ฒ Installation
Net is available through CocoaPods. To install it, simply add the following line to your Podfile:
pod 'NetClient'
For Swift 3 compatibility use:
pod 'NetClient', '~> 0.2'
Or you can install it with Carthage:
github "intelygenz/NetClient-iOS"
Or install it with Swift Package Manager:
dependencies: [
.package(url: "https://github.com/intelygenz/NetClient-iOS.git")
]
๐ Usage
Build a NetRequest
import Net
do {
let request = try NetRequest.builder("YOUR_URL")!
.setAccept(.json)
.setCache(.reloadIgnoringLocalCacheData)
.setMethod(.PATCH)
.setTimeout(20)
.setJSONBody(["foo", "bar"])
.setContentType(.json)
.setServiceType(.background)
.setCacheControls([.maxAge(500)])
.setURLParameters(["foo": "bar"])
.setAcceptEncodings([.gzip, .deflate])
.setContentEncodings([.gzip])
.setBasicAuthorization(user: "user", password: "password")
.setHeaders(["foo": "bar"])
.build()
} catch {
print("Request error: \(error)")
}
Request asynchronously
import Net
let net = NetURLSession()
net.data(URL(string: "YOUR_URL")!).async { (response, error) in
do {
if let object: [AnyHashable: Any] = try response?.object() {
print("Response dictionary: \(object)")
} else if let error = error {
print("Net error: \(error)")
}
} catch {
print("Parse error: \(error)")
}
}
Request synchronously
import Net
let net = NetURLSession()
do {
let object: [AnyHashable: Any] = try net.data("YOUR_URL").sync().object()
print("Response dictionary: \(object)")
} catch {
print("Error: \(error)")
}
Request from cache
import Net
let net = NetURLSession()
do {
let object: [AnyHashable: Any] = try net.data("YOUR_URL").cached().object()
print("Response dictionary: \(object)")
} catch {
print("Error: \(error)")
}
Track progress
import Net
let net = NetURLSession()
do {
let task = try net.data("YOUR_URL").progress({ progress in
print(progress)
}).sync()
} catch {
print("Error: \(error)")
}
Add interceptors for all requests
import Net
let net = NetURLSession()
net.addRequestInterceptor { request in
request.addHeader("foo", value: "bar")
request.setBearerAuthorization(token: "token")
return request
}
Retry requests
import Net
let net = NetURLSession()
net.retryClosure = { response, _, _ in response?.statusCode == XXX }
do {
let task = try net.data("YOUR_URL").retry({ response, error, retryCount in
return retryCount < 2
}).sync()
} catch {
print("Error: \(error)")
}
๐งโโ๏ธ Codable
Encodable
import Net
let request = NetRequest.builder("YOUR_URL")!
.setJSONObject(Encodable())
.build()
Decodable
import Net
let net = NetURLSession()
do {
let object: Decodable = try net.data("YOUR_URL").sync().decode()
print("Response object: \(object)")
} catch {
print("Error: \(error)")
}
๐ค Integrations
Love Alamofire?
pod 'NetClient/Alamofire'
import Net
let net = NetAlamofire()
...
Love Moya?
pod 'NetClient/Moya'
import Net
import Moya
let request = NetRequest("YOUR_URL")!
let provider = MoyaProvider<NetRequest>()
provider.request(request) { result in
switch result {
case let .success(response):
print("Response: \(response)")
case let .failure(error):
print("Error: \(error)")
}
}
Love Kommander?
pod 'NetClient/Kommander'
import Net
import Kommander
let net = NetURLSession()
let kommander = Kommander.default
net.data(URL(string: "YOUR_URL")!).execute(by: kommander, onSuccess: { object in
print("Response dictionary: \(object as [AnyHashable: Any])")
}) { error in
print("Error: \(String(describing: error?.localizedDescription))")
}
net.data(URL(string: "YOUR_URL")!).executeDecoding(by: kommander, onSuccess: { object in
print("Response object: \(object as Decodable)")
}) { error in
print("Error: \(String(describing: error?.localizedDescription))")
}
Love RxSwift?
pod 'NetClient/RxSwift'
import Net
import RxSwift
let request = NetRequest("YOUR_URL")!
_ = net.data(request).rx.response().observeOn(MainScheduler.instance).subscribe { print($0) }
Stub Implementation
pod 'NetClient/Stub'
import Net
let net = NetStub()
net.asyncBehavior = .delayed(.main, .seconds(10)) // If you want to delay the response.
net.nextResult = .response(NetResponse.builder()....build())
// Your test request here
net.nextResult = .error(.net(code: 500, message: "Your network error.", headers: ..., object: ..., underlying: ...))
// Your test request here
โค๏ธ Etc.
- Contributions are very welcome.
- Attribution is appreciated (let's spread the word!), but not mandatory.
๐จโ๐ป Authors
alexruperez, [email protected]
๐ฎโโ๏ธ License
Net is available under the MIT license. See the LICENSE file for more info.
*Note that all licence references and agreements mentioned in the NetClient README section above
are relevant to that project's source code only.