ConfigurableTableViewController alternatives and similar libraries
Based on the "Table View" category.
Alternatively, view ConfigurableTableViewController alternatives based on common mentions on social networks and blogs.
-
DZNEmptyDataSet
DISCONTINUED. A drop-in UITableView/UICollectionView superclass category for showing empty datasets whenever the view has no content to display -
folding-cell
:octocat: ๐ FoldingCell is an expanding content cell with animation made by @Ramotion -
SWTableViewCell
An easy-to-use UITableViewCell subclass that implements a swippable content view which exposes utility buttons (similar to iOS 7 Mail Application) -
MGSwipeTableCell
An easy to use UITableViewCell subclass that allows to display swippable buttons with a variety of transitions. -
CSStickyHeaderFlowLayout
UICollectionView replacement of UITableView. Do even more like Parallax Header, Sticky Section Header. Made for iOS 7. -
preview-transition
:octocat: PreviewTransition is a simple preview gallery UI controller with animated tranisitions. Swift UI library made by @Ramotion -
ParallaxTableViewHeader
Parallax scrolling effect on UITableView header view when a tableView is scrolled -
ZYThumbnailTableView
a TableView have thumbnail cell only, and you can use gesture let it expands other expansionView, all diy -
MEVFloatingButton
An iOS drop-in UITableView, UICollectionView and UIScrollView superclass category for showing a customizable floating button on top of it. -
AEAccordion
Simple and lightweight UITableViewController with accordion effect (expand / collapse cells) -
VBPiledView
Simple and beautiful stacked UIView to use as a replacement for an UITableView, UIImageView or as a menu -
CollapsableTableKit
DISCONTINUED. A kit for building tableviews with a collapsable animation, for each section.
InfluxDB - Power Real-Time Data Analytics at Scale
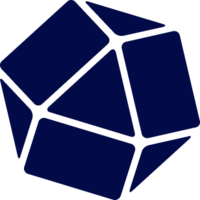
* Code Quality Rankings and insights are calculated and provided by Lumnify.
They vary from L1 to L5 with "L5" being the highest.
Do you think we are missing an alternative of ConfigurableTableViewController or a related project?
README
ConfigurableTableViewController
Simple view controller that provides a way to configure a table view with multiple types of cells while keeping type safety. To learn what and whys I encourage you to read the associated blog post.
Usage
Let's say we want to present a table view controller with four rows: two with text and two with images. We start by creating view data structures that cells will be configurable with:
struct TextCellViewData {
let title: String
}
struct ImageCellViewData {
let image: UIImage
}
and the cells themselves:
class TextTableViewCell: UITableViewCell {
func updateWithViewData(viewData: TextCellViewData) {
textLabel?.text = viewData.title
}
}
class ImageTableViewCell: UITableViewCell {
func updateWithViewData(viewData: ImageCellViewData) {
imageView?.image = viewData.image
}
}
Now to present a table view controller with those cells, we simply configure it in the following way:
import ConfigurableTableViewController
...
let viewController = ConfigurableTableViewController(items: [
CellConfigurator<TextTableViewCell>(viewData: TextCellViewData(title: "Foo")),
CellConfigurator<ImageTableViewCell>(viewData: ImageCellViewData(image: apple)),
CellConfigurator<ImageTableViewCell>(viewData: ImageCellViewData(image: google)),
CellConfigurator<TextTableViewCell>(viewData: TextCellViewData(title: "Bar")),
])
presentViewController(viewController, animated: true, completion: nil)
And ta-da :balloon::
I encourage you to check both the implementation and an example app.
Demo
To run the example project; clone the repo, open the project and run ExampleApp
target.
Requirements
iOS 8 and above.
Author
Arkadiusz Holko: