CameraManager alternatives and similar libraries
Based on the "Camera" category.
Alternatively, view CameraManager alternatives based on common mentions on social networks and blogs.
-
SCRecorder
iOS camera engine with Vine-like tap to record, animatable filters, slow motion, segments editing -
PBJVision
DISCONTINUED. πΈ iOS Media Capture β features touch-to-record video, slow motion, and photography -
ALCameraViewController
DISCONTINUED. A camera view controller with custom image picker and image cropping. -
TGCameraViewController
DISCONTINUED. Custom camera with AVFoundation. Beautiful, light and easy to integrate with iOS projects. -
Cool-iOS-Camera
A fully customisable and modern camera implementation for iOS made with AVFoundation. -
Lumina
A camera designed in Swift for easily integrating CoreML models - as well as image streaming, QR/Barcode detection, and many other features -
RSBarcodes_Swift
1D and 2D barcodes reader and generators for iOS 8 with delightful controls. Now Swift. -
CameraEngine
DISCONTINUED. ππ· Camera engine for iOS, written in Swift, above AVFoundation. π -
CameraKit-iOS
Library for iOS Camera API. Massively increase performance and ease of use within your next iOS Project. -
JVTImageFilePicker
Easy and beautiful way for a user to pick content, files or images. Written in Objective C
InfluxDB - Power Real-Time Data Analytics at Scale
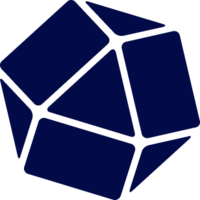
* Code Quality Rankings and insights are calculated and provided by Lumnify.
They vary from L1 to L5 with "L5" being the highest.
Do you think we are missing an alternative of CameraManager or a related project?
README
Camera Manager
This is a simple Swift class to provide all the configurations you need to create custom camera view in your app. It follows orientation change and updates UI accordingly, supports front and rear camera selection, pinch to zoom, tap to focus, exposure slider, different flash modes, inputs and outputs and QRCode detection. Just drag, drop and use.
We've also written a blog post about it. You can read it here.
Installation with CocoaPods
The easiest way to install the CameraManager is with CocoaPods
Podfile
use_frameworks!
pod 'CameraManager', '~> 5.1'
Installation with Swift Package Manager
The Swift Package Manager is a tool for managing the distribution of Swift code.
Add CameraManager
as a dependency in your Package.swift
file:
import PackageDescription
let package = Package(
dependencies: [
.Package(url: "https://github.com/imaginary-cloud/CameraManager", from: "5.1.3")
]
)
Installation with Carthage
Carthage is another dependency management tool written in Swift.
Add the following line to your Cartfile:
github "imaginary-cloud/CameraManager" >= 5.1
And run carthage update
to build the dynamic framework.
How to use
To use it you just add the preview layer to your desired view, you'll get back the state of the camera if it's unavailable, ready or the user denied access to it. Have in mind that in order to retain the AVCaptureSession you will need to retain cameraManager instance somewhere, ex. as an instance constant.
let cameraManager = CameraManager()
cameraManager.addPreviewLayerToView(self.cameraView)
To shoot image all you need to do is call:
cameraManager.capturePictureWithCompletion({ result in
switch result {
case .failure:
// error handling
case .success(let content):
self.myImage = content.asImage;
}
})
To record video you call:
cameraManager.startRecordingVideo()
cameraManager.stopVideoRecording({ (videoURL, recordError) -> Void in
guard let videoURL = videoURL else {
//Handle error of no recorded video URL
}
do {
try FileManager.default.copyItem(at: videoURL, to: self.myVideoURL)
}
catch {
//Handle error occured during copy
}
})
To zoom in manually:
let zoomScale = CGFloat(2.0)
cameraManager.zoom(zoomScale)
Properties
You can set input device to front or back camera. (Default: .Back)
cameraManager.cameraDevice = .front || .back
You can specify if the front camera image should be horizontally fliped. (Default: false)
cameraManager.shouldFlipFrontCameraImage = true || false
You can enable or disable gestures on camera preview. (Default: true)
cameraManager.shouldEnableTapToFocus = true || false
cameraManager.shouldEnablePinchToZoom = true || false
cameraManager.shouldEnableExposure = true || false
You can set output format to Image, video or video with audio. (Default: .stillImage)
cameraManager.cameraOutputMode = .stillImage || .videoWithMic || .videoOnly
You can set the quality based on the AVCaptureSession.Preset values (Default: .high)
cameraManager.cameraOutputQuality = .low || .medium || .high || *
*
check all the possible values here
You can also check if you can set a specific preset value:
if .cameraManager.canSetPreset(preset: .hd1280x720) {
cameraManager.cameraOutputQuality = .hd1280x720
} else {
cameraManager.cameraOutputQuality = .high
}
You can specify the focus mode. (Default: .continuousAutoFocus)
cameraManager.focusMode = .autoFocus || .continuousAutoFocus || .locked
You can specifiy the exposure mode. (Default: .continuousAutoExposure)
cameraManager.exposureMode = .autoExpose || .continuousAutoExposure || .locked || .custom
You can change the flash mode (it will also set corresponding flash mode). (Default: .off)
cameraManager.flashMode = .off || .on || .auto
You can specify the stabilisation mode to be used during a video record session. (Default: .auto)
cameraManager.videoStabilisationMode = .auto || .cinematic
You can get the video stabilization mode currently active. If video stabilization is neither supported or active it will return .off
.
cameraManager.activeVideoStabilisationMode
You can enable location services for storing GPS location when saving to Camera Roll. (Default: false)
cameraManager.shouldUseLocationServices = true || false
In case you use location it's mandatory to add NSLocationWhenInUseUsageDescription
key to the Info.plist
in your app. More Info
For getting the gps location when calling capturePictureWithCompletion
you should use the CaptureResult
as data
(see Example App).
You can specify if you want to save the files to phone library. (Default: true)
cameraManager.writeFilesToPhoneLibrary = true || false
You can specify the album names for image and video recordings.
cameraManager.imageAlbumName = "Image Album Name"
cameraManager.videoAlbumName = "Video Album Name"
You can specify if you want to disable animations. (Default: true)
cameraManager.animateShutter = true || false
cameraManager.animateCameraDeviceChange = true || false
You can specify if you want the user to be asked about camera permissions automatically when you first try to use the camera or manually. (Default: true)
cameraManager.showAccessPermissionPopupAutomatically = true || false
To check if the device supports flash call:
cameraManager.hasFlash
To change flash mode to the next available one you can use this handy function which will also return current value for you to update the UI accordingly:
cameraManager.changeFlashMode()
You can even setUp your custom block to handle error messages: It can be customized to be presented on the Window root view controller, for example.
cameraManager.showErrorBlock = { (erTitle: String, erMessage: String) -> Void in
var alertController = UIAlertController(title: erTitle, message: erMessage, preferredStyle: .alert)
alertController.addAction(UIAlertAction(title: "OK", style: UIAlertAction.Style.default, handler: { (alertAction) -> Void in
}))
let topController = UIApplication.shared.keyWindow?.rootViewController
if (topController != nil) {
topController?.present(alertController, animated: true, completion: { () -> Void in
//
})
}
}
You can set if you want to detect QR codes:
cameraManager.startQRCodeDetection { (result) in
switch result {
case .success(let value):
print(value)
case .failure(let error):
print(error.localizedDescription)
}
}
and don't forget to call cameraManager.stopQRCodeDetection()
whenever you done detecting.
Support
Supports iOS 9 and above. Xcode 11.4 is required to build the latest code written in Swift 5.2.
Now it's compatible with latest Swift syntax, so if you're using any Swift version prior to 5 make sure to use one of the previously tagged releases:
License
Copyright Β© 2010-2020 Imaginary Cloud. This library is licensed under the MIT license.
About Imaginary Cloud
At Imaginary Cloud, we build world-class web & mobile apps. Our Front-end developers and UI/UX designers are ready to create or scale your digital product. Take a look at our website and get in touch! We'll take it from there.
*Note that all licence references and agreements mentioned in the CameraManager README section above
are relevant to that project's source code only.