Anchorage alternatives and similar libraries
Based on the "Layout" category.
Alternatively, view Anchorage alternatives based on common mentions on social networks and blogs.
-
Masonry
Harness the power of AutoLayout NSLayoutConstraints with a simplified, chainable and expressive syntax. Supports iOS and OSX Auto Layout -
PureLayout
The ultimate API for iOS & OS X Auto Layout — impressively simple, immensely powerful. Objective-C and Swift compatible. -
MyLinearLayout
MyLayout is a powerful iOS UI framework implemented by Objective-C. It integrates the functions with Android Layout,iOS AutoLayout,SizeClass, HTML CSS float and flexbox and bootstrap. So you can use LinearLayout,RelativeLayout,FrameLayout,TableLayout,FlowLayout,FloatLayout,PathLayout,GridLayout,LayoutSizeClass to build your App 自动布局 UIView UITableView UICollectionView RTL -
PinLayout
Fast Swift Views layouting without auto layout. No magic, pure code, full control and blazing fast. Concise syntax, intuitive, readable & chainable. [iOS/macOS/tvOS/CALayer] -
FlexLayout
FlexLayout adds a nice Swift interface to the highly optimized facebook/yoga flexbox implementation. Concise, intuitive & chainable syntax. -
Luminous
Luminous provides you a lot of information about the system and a lot of handy methods to quickly get useful data on the iOS platform. -
MisterFusion
MisterFusion is Swift DSL for AutoLayout. It is the extremely clear, but concise syntax, in addition, can be used in both Swift and Objective-C. Support Safe Area and Size Class. -
ManualLayout
✂ Easy to use and flexible library for manually laying out views and layers for iOS and tvOS. Supports AsyncDisplayKit. -
QuickLayout
Written in pure Swift, QuickLayout offers a simple and easy way to manage Auto Layout in code. -
MondrianLayout
🏗 A way to build AutoLayout rapidly than using InterfaceBuilder(XIB, Storyboard) in iOS. -
Framezilla
DISCONTINUED. Elegant library that wraps working with frames with a nice chaining syntax. -
BBLocationManager
A Location Manager for easily implementing location services & geofencing in iOS. Ready for iOS 11.
InfluxDB - Power Real-Time Data Analytics at Scale
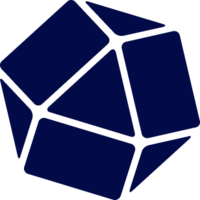
* Code Quality Rankings and insights are calculated and provided by Lumnify.
They vary from L1 to L5 with "L5" being the highest.
Do you think we are missing an alternative of Anchorage or a related project?
README
Anchorage
A lightweight collection of intuitive operators and utilities that simplify Auto Layout code. Anchorage is built directly on top of the NSLayoutAnchor
API.
Each expression acts on one or more NSLayoutAnchor
s, and returns active NSLayoutConstraint
s. If you want inactive constraints, here's how to do that.
Usage
Alignment
// Pin the button to 12 pt from the leading edge of its container
button.leadingAnchor == container.leadingAnchor + 12
// Pin the button to at least 12 pt from the trailing edge of its container
button.trailingAnchor <= container.trailingAnchor - 12
// Center one or both axes of a view
button.centerXAnchor == container.centerXAnchor
button.centerAnchors == container.centerAnchors
Relative Alignment
// Position a view to be centered at 2/3 of its container's width
view.centerXAnchor == 2 * container.trailingAnchor / 3
// Pin the top of a view at 25% of container's height
view.topAnchor == container.bottomAnchor / 4
Sizing
// Constrain a view's width to be at most 100 pt
view.widthAnchor <= 100
// Constraint a view to a fixed size
imageView.sizeAnchors == CGSize(width: 100, height: 200)
// Constrain two views to be the same size
imageView.sizeAnchors == view.sizeAnchors
// Constrain view to 4:3 aspect ratio
view.widthAnchor == 4 * view.heightAnchor / 3
Composite Anchors
Constrain multiple edges at a time with this syntax:
// Constrain the leading, trailing, top and bottom edges to be equal
imageView.edgeAnchors == container.edgeAnchors
// Inset the edges of a view from another view
let insets = UIEdgeInsets(top: 5, left: 10, bottom: 15, right: 20)
imageView.edgeAnchors == container.edgeAnchors + insets
// Inset the leading and trailing anchors by 10
imageView.horizontalAnchors >= container.horizontalAnchors + 10
// Inset the top and bottom anchors by 10
imageView.verticalAnchors >= container.verticalAnchors + 10
Use leading and trailing
Using leftAnchor
and rightAnchor
is rarely the right choice. To encourage this, horizontalAnchors
and edgeAnchors
use the leadingAnchor
and trailingAnchor
layout anchors.
Inset instead of Shift
When constraining leading/trailing or top/bottom, it is far more common to work in terms of an inset from the edges instead of shifting both edges in the same direction. When building the expression, Anchorage will flip the relationship and invert the constant in the constraint on the far side of the axis. This makes the expressions much more natural to work with.
Priority
The ~
is used to specify priority of the constraint resulting from any Anchorage expression:
// Align view 20 points from the center of its superview, with system-defined low priority
view.centerXAnchor == view.superview.centerXAnchor + 20 ~ .low
// Align view 20 points from the center of its superview, with (required - 1) priority
view.centerXAnchor == view.superview.centerXAnchor + 20 ~ .required - 1
// Align view 20 points from the center of its superview, with custom priority
view.centerXAnchor == view.superview.centerXAnchor + 20 ~ 752
The layout priority is an enum with the following values:
.required
-UILayoutPriorityRequired
(default).high
-UILayoutPriorityDefaultHigh
.low
-UILayoutPriorityDefaultLow
.fittingSize
-UILayoutPriorityFittingSizeLevel
Storing Constraints
To store constraints created by Anchorage, simply assign the expression to a variable:
// A single (active) NSLayoutConstraint
let topConstraint = (imageView.topAnchor == container.topAnchor)
// EdgeConstraints represents a collection of constraints
// You can retrieve the NSLayoutConstraints individually,
// or get an [NSLayoutConstraint] via .all, .horizontal, or .vertical
let edgeConstraints = (button.edgeAnchors == container.edgeAnchors).all
Batching Constraints
By default, Anchorage returns active layout constraints. If you'd rather return inactive constraints for use with the NSLayoutConstraint.activate(_:)
method for performance reasons, you can do it like this:
let constraints = Anchorage.batch(active: false) {
view1.widthAnchor == view2.widthAnchor
view1.heightAnchor == view2.heightAnchor / 2 ~ .low
// ... as many constraints as you want
}
// Later:
NSLayoutConstraint.activate(constraints)
You can also pass active: true
if you want the constraints in the array to be automatically activated in a batch.
Autoresizing Mask
Anchorage sets the translatesAutoresizingMaskIntoConstraints
property to false
on the left hand side of the expression, so you should never need to set this property manually. This is important to be aware of in case the container view relies on translatesAutoresizingMaskIntoConstraints
being set to true
. We tend to keep child views on the left hand side of the expression to avoid this problem, especially when constraining to a system-supplied view.
A Note on Compile Times
Anchorage overloads a few Swift operators, which can lead to increased compile times. You can reduce this overhead by surrounding these operators with /
, like so:
Operator | Faster Alternative |
---|---|
== |
/==/ |
<= |
/<=/ |
>= |
/>=/ |
For example, view1.edgeAnchors == view2.edgeAnchors
would become view1.edgeAnchors /==/ view2.edgeAnchors
.
Installation
CocoaPods
To integrate Anchorage into your Xcode project using CocoaPods, specify it in your Podfile:
pod 'Anchorage'
Carthage
To integrate Anchorage into your Xcode project using Carthage, specify it in your Cartfile:
github "Rightpoint/Anchorage"
Run carthage update
to build the framework and drag the built
Anchorage.framework
into your Xcode project.
License
This code and tool is under the MIT License. See LICENSE
file in this repository.
Any ideas and contributions welcome!
*Note that all licence references and agreements mentioned in the Anchorage README section above
are relevant to that project's source code only.