Swiftstraints alternatives and similar libraries
Based on the "Layout" category.
Alternatively, view Swiftstraints alternatives based on common mentions on social networks and blogs.
-
Masonry
Harness the power of AutoLayout NSLayoutConstraints with a simplified, chainable and expressive syntax. Supports iOS and OSX Auto Layout -
PureLayout
The ultimate API for iOS & OS X Auto Layout — impressively simple, immensely powerful. Objective-C and Swift compatible. -
MyLinearLayout
MyLayout is a powerful iOS UI framework implemented by Objective-C. It integrates the functions with Android Layout,iOS AutoLayout,SizeClass, HTML CSS float and flexbox and bootstrap. So you can use LinearLayout,RelativeLayout,FrameLayout,TableLayout,FlowLayout,FloatLayout,PathLayout,GridLayout,LayoutSizeClass to build your App 自动布局 UIView UITableView UICollectionView RTL -
PinLayout
Fast Swift Views layouting without auto layout. No magic, pure code, full control and blazing fast. Concise syntax, intuitive, readable & chainable. [iOS/macOS/tvOS/CALayer] -
FlexLayout
FlexLayout adds a nice Swift interface to the highly optimized facebook/yoga flexbox implementation. Concise, intuitive & chainable syntax. -
Luminous
Luminous provides you a lot of information about the system and a lot of handy methods to quickly get useful data on the iOS platform. -
MisterFusion
MisterFusion is Swift DSL for AutoLayout. It is the extremely clear, but concise syntax, in addition, can be used in both Swift and Objective-C. Support Safe Area and Size Class. -
ManualLayout
✂ Easy to use and flexible library for manually laying out views and layers for iOS and tvOS. Supports AsyncDisplayKit. -
QuickLayout
Written in pure Swift, QuickLayout offers a simple and easy way to manage Auto Layout in code. -
MondrianLayout
🏗 A way to build AutoLayout rapidly than using InterfaceBuilder(XIB, Storyboard) in iOS. -
Framezilla
DISCONTINUED. Elegant library that wraps working with frames with a nice chaining syntax. -
BBLocationManager
A Location Manager for easily implementing location services & geofencing in iOS. Ready for iOS 11.
WorkOS - The modern identity platform for B2B SaaS
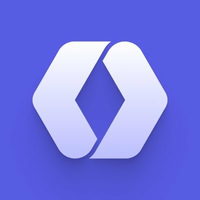
* Code Quality Rankings and insights are calculated and provided by Lumnify.
They vary from L1 to L5 with "L5" being the highest.
Do you think we are missing an alternative of Swiftstraints or a related project?
README
Swiftstraints
Swiftstraints
can turn verbose auto-layout code:
let constraint = NSLayoutConstraint(item: blueView,
attribute: NSLayoutAttribute.Width,
relatedBy: NSLayoutRelation.Equal,
toItem: redView,
attribute: NSLayoutAttribute.Width,
multiplier: 1.0,
constant: 0.0)
Into one just one line of code:
let constraint = blueView.widthAnchor == redView.widthAnchor
Or transform your less than consise visual format language code:
let constraints = NSLayoutConstraint.constraintsWithVisualFormat("H:|[leftView]-10-[rightView]|",
options: NSLayoutFormatOptions(0),
metrics: nil,
views: ["leftView":leftView, "rightView":rightView])
Into the following:
let constraints = NSLayoutConstraints("H:|[\(leftView)]-10-[\(rightView)]|")
That was easy!
Installation
Swiftstraints
is available through CocoaPods. To install, simply include the following lines in your podfile:
use_frameworks!
pod 'Swiftstraints'
Be sure to import the module at the top of your .swift files:
import Swiftstraints
Alternatively, clone this repo or download it as a zip and include the classes in your project.
Constraints
With Swiftstraints
you can create constraints that look just Apple's generic constraint definition:
item1.attribute1 = multiplier × item2.attribute2 + constant
Swifstraints
utilizes the new layout anchors introduced in iOS 9:
let view = UIView()
view.widthAnchor
view.heightAnchor
view.trailingAnchor
view.centerXAnchor
etc...
Swiftstraints
implements operator overloading so that you can easily create custom constraints:
let blueView = UIView()
let redView = UIView()
let constraint = blueView.heightAnchor == redView.heightAnchor
Just as you would expect, you can specify a multiplier:
let constraint = blueView.heightAnchor == 2.0 * redView.heightAnchor
Or add a constant:
let constraint = blueView.heightAnchor == redView.heightAnchor + 10.0
You can specify inequalities:
let constraint = blueView.heightAnchor <= redView.heightAnchor
And you can define constant constraints if you so choose:
let constraint = blueView.heightAnchor == 100.0
Swiftstraints can readily compute relatively complex constraints:
let constraint = blueView.heightAnchor * 1.4 - 5.0 >= redView.heightAnchor / 3.0 + 400
It's really easy.
Visual Format Language
Apple provides an API that lets you create multiple constraints simultaneously with the Visual Format Language. As we saw before it can be a little cumbersome:
let constraints = NSLayoutConstraint.constraintsWithVisualFormat("H:|[leftView]-10-[rightView]|",
options: NSLayoutFormatOptions(0),
metrics: nil,
views: ["leftView":leftView, "rightView":rightView])
Swiftstraints
uses string interpolation to let you specify the same constraints in one line of code:
let constraints = NSLayoutConstraints("H:|[\(leftView)]-10-[\(rightView)]|")
Swiftstraints
also extends UIView
so that you can add constraints easily using the interpolated string format:
superview.addConstraints("H:|[\(leftView)]-10-[\(rightView)]|")
Super easy, super simple.
Revision History
- 3.0.1 - Bug fixes and limited iOS 8 support (Thank you catjia1011)
- 3.0.0 - Updated to Swift 3
- 2.2.0 - Added support for UILayoutPriority
- 2.1.0 - Fixed a view reference bug and added a new convenience method for adding constraints
- 2.0.2 - Added support for tvOS target.
- 2.0.1 - Updated to include support for axis anchors, increased test coverage and more documentation.
- 2.0.0 - Updated for Swift 2.0 and iOS 9. Now uses layout anchors for simple constraints and string interpolation for Visual Format Language constraints.
- 1.1.0 - Minor API tweaks
- 1.0.0 - Initial Release
Author
Brad Hilton, [email protected]
License
Swiftstraints is available under the MIT license. See the LICENSE file for more info.
*Note that all licence references and agreements mentioned in the Swiftstraints README section above
are relevant to that project's source code only.