RxAutomaton alternatives and similar libraries
Based on the "Reactive Programming" category.
Alternatively, view RxAutomaton alternatives based on common mentions on social networks and blogs.
-
OpenCombine
Open source implementation of Apple's Combine framework for processing values over time. -
Tokamak
DISCONTINUED. SwiftUI-compatible framework for building browser apps with WebAssembly and native apps for other platforms [Moved to: https://github.com/TokamakUI/Tokamak] -
Interstellar
DISCONTINUED. Simple and lightweight Functional Reactive Coding in Swift for the rest of us. :large_orange_diamond: -
Verge
đŁ A robust Swift state-management framework designed for complex applications, featuring an integrated ORM for efficient data handling. -
VueFlux
:recycle: Unidirectional State Management Architecture for Swift - Inspired by Vuex and Flux -
RxReduce
DISCONTINUED. Lightweight framework that ease the implementation of a state container pattern in a Reactive Programming compliant way. -
LightweightObservable
đŹ A lightweight implementation of an observable sequence that you can subscribe to. -
ReactiveArray
An array class implemented in Swift that can be observed using ReactiveCocoa's Signals -
STDevRxExt
STDevRxExt contains some extension functions for RxSwift and RxCocoa which makes our live easy. -
RxAlamoRecord
RxAlamoRecord combines the power of the AlamoRecord and RxSwift libraries to create a networking layer that makes interacting with API's easier than ever reactively.
WorkOS - The modern identity platform for B2B SaaS
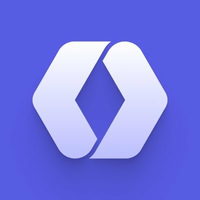
* Code Quality Rankings and insights are calculated and provided by Lumnify.
They vary from L1 to L5 with "L5" being the highest.
Do you think we are missing an alternative of RxAutomaton or a related project?
README
RxAutomaton
RxSwift port of ReactiveAutomaton (State Machine).
Terminology
Whenever the word "signal" or "(signal) producer" appears (derived from ReactiveCocoa), they mean "hot-observable" and "cold-observable".
Example
(Demo app is bundled in the project)
[](Assets/login-diagram.png)
To make a state transition diagram like above with additional effects, follow these steps:
// 1. Define `State`s and `Input`s.
enum State {
case loggedOut, loggingIn, loggedIn, loggingOut
}
enum Input {
case login, loginOK, logout, logoutOK
case forceLogout
}
// Additional effects (`Observable`s) while state-transitioning.
// (NOTE: Use `Observable.empty()` for no effect)
let loginOKProducer = /* show UI, setup DB, request APIs, ..., and send `Input.loginOK` */
let logoutOKProducer = /* show UI, clear cache, cancel APIs, ..., and send `Input.logoutOK` */
let forcelogoutOKProducer = /* do something more special, ..., and send `Input.logoutOK` */
let canForceLogout: State -> Bool = [.loggingIn, .loggedIn].contains
// 2. Setup state-transition mappings.
let mappings: [Automaton<State, Input>.EffectMapping] = [
/* Input | fromState => toState | Effect */
/* ----------------------------------------------------------------*/
.login | .loggedOut => .loggingIn | loginOKProducer,
.loginOK | .loggingIn => .loggedIn | .empty(),
.logout | .loggedIn => .loggingOut | logoutOKProducer,
.logoutOK | .loggingOut => .loggedOut | .empty(),
.forceLogout | canForceLogout => .loggingOut | forceLogoutOKProducer
]
// 3. Prepare input pipe for sending `Input` to `Automaton`.
let (inputSignal, inputObserver) = Observable<Input>.pipe()
// 4. Setup `Automaton`.
let automaton = Automaton(
state: .loggedOut,
input: inputSignal,
mapping: reduce(mappings), // combine mappings using `reduce` helper
strategy: .latest // NOTE: `.latest` cancels previous running effect
)
// Observe state-transition replies (`.success` or `.failure`).
automaton.replies.subscribe(next: { reply in
print("received reply = \(reply)")
})
// Observe current state changes.
automaton.state.asObservable().subscribe(next: { state in
print("current state = \(state)")
})
And let's test!
let send = inputObserver.onNext
expect(automaton.state.value) == .loggedIn // already logged in
send(Input.logout)
expect(automaton.state.value) == .loggingOut // logging out...
// `logoutOKProducer` will automatically send `Input.logoutOK` later
// and transit to `State.loggedOut`.
expect(automaton.state.value) == .loggedOut // already logged out
send(Input.login)
expect(automaton.state.value) == .loggingIn // logging in...
// `loginOKProducer` will automatically send `Input.loginOK` later
// and transit to `State.loggedIn`.
// đ¨đ˝ < But wait, there's more!
// Let's send `Input.forceLogout` immediately after `State.loggingIn`.
send(Input.forceLogout) // đĽđŁđĽ
expect(automaton.state.value) == .loggingOut // logging out...
// `forcelogoutOKProducer` will automatically send `Input.logoutOK` later
// and transit to `State.loggedOut`.
License
[MIT](LICENSE)
*Note that all licence references and agreements mentioned in the RxAutomaton README section above
are relevant to that project's source code only.