RxMediaPicker alternatives and similar libraries
Based on the "Reactive Programming" category.
Alternatively, view RxMediaPicker alternatives based on common mentions on social networks and blogs.
-
OpenCombine
Open source implementation of Apple's Combine framework for processing values over time. -
Tokamak
DISCONTINUED. SwiftUI-compatible framework for building browser apps with WebAssembly and native apps for other platforms [Moved to: https://github.com/TokamakUI/Tokamak] -
Interstellar
DISCONTINUED. Simple and lightweight Functional Reactive Coding in Swift for the rest of us. :large_orange_diamond: -
Verge
🟣 A robust Swift state-management framework designed for complex applications, featuring an integrated ORM for efficient data handling. -
VueFlux
:recycle: Unidirectional State Management Architecture for Swift - Inspired by Vuex and Flux -
RxReduce
DISCONTINUED. Lightweight framework that ease the implementation of a state container pattern in a Reactive Programming compliant way. -
LightweightObservable
📬 A lightweight implementation of an observable sequence that you can subscribe to. -
ReactiveArray
An array class implemented in Swift that can be observed using ReactiveCocoa's Signals -
STDevRxExt
STDevRxExt contains some extension functions for RxSwift and RxCocoa which makes our live easy. -
RxAlamoRecord
RxAlamoRecord combines the power of the AlamoRecord and RxSwift libraries to create a networking layer that makes interacting with API's easier than ever reactively.
WorkOS - The modern identity platform for B2B SaaS
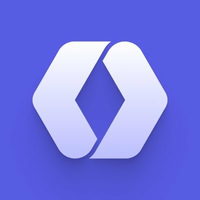
* Code Quality Rankings and insights are calculated and provided by Lumnify.
They vary from L1 to L5 with "L5" being the highest.
Do you think we are missing an alternative of RxMediaPicker or a related project?
README
RxMediaPicker
RxMediaPicker is a RxSwift wrapper built around UIImagePickerController consisting in a simple interface for common actions like picking photos or videos stored on the device, recording a video or taking a photo.
If you ever used UIImagePickerController you know how it can become quite verbose and introduce some unnecessary complexity, as you use the same class to do everything, from picking photos or videos from the library, recording videos, etc. At the same time, you have all these different properties and methods which can be used when you are dealing with photos, others for when you're dealing with videos, and others in all cases.
If you're interested, check the resulting article RxMediaPicker — picking photos and videos the cool kids’ way!
Note: As RxMediaPicker is still in its early days, the interface may change in future versions.
Features
- [x] Reactive wrapper built around UIImagePickerController.
- [x] Provides an interface for common operations like picking photos from the library, recording videos, etc.
- [x] Handles edited videos properly - something UIImagePickerController doesn't do for you!
- [x] Decouples the typical UIImagePickerController boilerplate from your code.
- [x] Reduces the complexity when compared to UIImagePickerController.
- [x] Easy to integrate and reuse accross your app.
Example
For a more complete example please check the demo app included (ideally run it on a device). This is how you would record a video using the camera:
import RxMediaPicker
import RxSwift
var picker: RxMediaPicker!
let disposeBag = DisposeBag()
override func viewDidLoad() {
super.viewDidLoad()
picker = RxMediaPicker(delegate: self)
}
func recordVideo() {
picker.recordVideo(maximumDuration: 10, editable: true)
.observeOn(MainScheduler.instance)
.subscribe(onNext: { url in
// Do something with the video url obtained!
}, onError: { error in
print("Error occurred!")
}, onCompleted: {
print("Completed")
}, onDisposed: {
print("Disposed")
})
.disposed(by: disposeBag)
}
Usage
Available operations
Based on their names, the operations available on RxMediaPicker should be self-explanatory. You can record a video, or pick an existing one stored on the device, and the same thing happens for photos. The only thing to note here is that picking a video will get you the video url, and picking a photo will get you a tuple consisting in the original image and an optional edited image (if any edits were made).
func recordVideo(device: UIImagePickerController.CameraDevice = .rear,
quality: UIImagePickerController.QualityType = .typeMedium,
maximumDuration: TimeInterval = 600,
editable: Bool = false) -> Observable<URL>
func selectVideo(source: UIImagePickerController.SourceType = .photoLibrary,
maximumDuration: TimeInterval = 600,
editable: Bool = false) -> Observable<URL>
func takePhoto(device: UIImagePickerController.CameraDevice = .rear,
flashMode: UIImagePickerController.CameraFlashMode = .auto,
editable: Bool = false) -> Observable<(UIImage, UIImage?)>
func selectImage(source: UIImagePickerController.SourceType = .photoLibrary,
editable: Bool = false) -> Observable<(UIImage, UIImage?)>
RxMediaPickerDelegate
func present(picker: UIImagePickerController)
func dismiss(picker: UIImagePickerController)
To be able to use RxMediaPicker you will need to adopt the protocol RxMediaPickerDelegate. This is required to indicate RxMediaPicker how the camera/photos picker should be presented. For example, you may want to present the photos library picker in a popover on the iPad, and use the entire screen on the iPhone.
Requirements
- iOS 8.0+
- Xcode 7.0+
Instalation
CocoaPods
CocoaPods is a dependency manager for Cocoa projects. You can install it with the following command:
$ gem install cocoapods
To integrate RxMediaPicker into your Xcode project using CocoaPods, include this in your Podfile:
platform :ios, '8.0'
use_frameworks!
pod 'RxMediaPicker'
Carthage
Carthage is a decentralized dependency manager that builds your dependencies and provides you with binary frameworks.
You can install Carthage with Homebrew using the following command:
$ brew update
$ brew install carthage
To integrate RxMediaPicker into your Xcode project using Carthage, specify it in your Cartfile
:
github "RxSwiftCommunity/RxMediaPicker" "master"
Run carthage
to build the framework and drag the built RxMediaPicker.framework
into your Xcode project.
Credits
Owned and maintained by Rui Costa (@ruipfcosta).
Contributing
Bug reports and pull requests are welcome.
License
RxMediaPicker is released under the MIT license. See LICENSE for details.
*Note that all licence references and agreements mentioned in the RxMediaPicker README section above
are relevant to that project's source code only.